Tutorial about using ui::ListView in cocos2dx
ListView is reuse object if objects go outside.
Let's learn basic usage about ui::ListView.
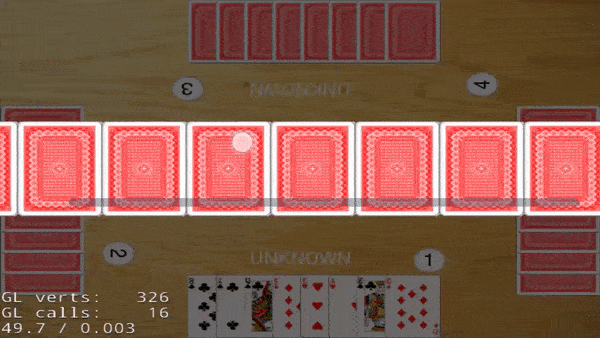
In this example ListView only contains Sprite class
and scroll horizontally and touch event
create and attach touch listener to ListView
#include "ui/CocosGui.h"
// create ListView
ListView::cardListView = ListView::create();
// set size
cardListView->setContentSize(Size(640,LIST_TRUMP_HEIGHT));
cardListView->setAnchorPoint(Vec2(0.5,0.5));
// put center
cardListView->setPosition(Vec2(DISPLAY_WIDTH / 2,DISPLAY_HEIGHT / 2));
// 1.set direction
cardListView->setDirection(ScrollView::Direction::HORIZONTAL);
// 2.touch callback
ui::ListView::ccListViewCallback callBackfunc = [this](cocos2d::Ref* senderRef, cocos2d::ui::ListView::EventType e) {
if(e == ListView::EventType::ON_SELECTED_ITEM_START){
}
else if (e == ListView::EventType::ON_SELECTED_ITEM_END) {
auto listView = static_cast(senderRef);
auto selectedIndex = listView->getCurSelectedIndex();
// access ListView item
Vec2 position = listView->getItem(selectedIndex)->getWorldPosition();
}
};
cardListView->addEventListener(callBackfunc);
this->addChild(cardListView);
1.setDirection
In this example use horizontal mode.
In DIRECTION enum
setDirection(VERTICAL) if want to.
enum class Direction
{
NONE,
VERTICAL,
HORIZONTAL,
BOTH
};
2.touch callBack
In this code set callback function in case touch list item.
ListView can detect if touchStart and touchEnd in EventType
enum class EventType
{
ON_SELECTED_ITEM_START,
ON_SELECTED_ITEM_END
};
Add and remove item
Let's see add and remove item example.
// add item to listView
for(int i = 0;i < MAX_TRUMP_NUM;++i) {
cocos2d::Sprite *trump = Sprite::createWithTexture(_trumpNode->getTexture(),cocos2d::Rect(RED_TRUMP_X,RED_TRUMP_Y,TRUMP_WIDTH,TRUMP_HEIGHT));
trump->setAnchorPoint(Vec2::ZERO);
trump->setScale(1.5, 1.5);
// container
ui::Layout* list_layout = ui::Layout::create();
list_layout->addChild(trump);
list_layout->setContentSize(Size(LIST_TRUMP_WIDTH,LIST_TRUMP_HEIGHT));
// need
list_layout->setTouchEnabled(true);
// push item
cardListView->pushBackCustomItem(list_layout);
}
}
In above example,create Sprite and add ui::Layout class.
and push by pushBackCustomItem function
To push widget class in listView show items
Remove item in ListView
To remove items use removeItem(int index)
To all removeAllItems()
This is available in cocos2dx 3.15.1